Javascript, NodeJS, Tools
10 Advanced Ways to Log to the Console Besides console.log
fetch("/posts/10-advanced-ways-to-log-to-the-console-besides-console-log")
In this article, we'll look at 10 advanced ways to output information to the console that will help you improve your code and speed up javascript code development and debugging.
When you need to debug something on the frontend, you use console.log. One of the problems with using console.log can be the "cluttering" of the console, which makes it difficult to read. Below, we will discuss 10 ways to improve logging to the console:
1. console.table
Instead of simply outputting an array or object, console.table displays the data in a tabular format, making it easier to read.
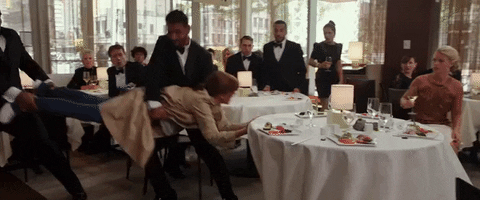
// Output an array of objects as a table
const users = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Doe' }
];
console.table(users);
This will output the array of users in a tabular format, with the properties of each object as columns and objects as rows.
2. console.group
console.group and console.groupEnd allow you to create nested collapsible groups in the console. This can be useful for organizing and structuring debugging input data, especially at different levels of nesting.
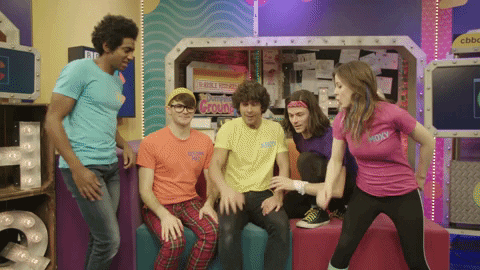
console.group('User Details');
console.log('Name: John Doe');
console.log('Age: 32');
console.groupEnd();
3. console.time
The console.time and console.timeEnd methods allow you to measure the time taken to execute a block of code. This can be useful for identifying and optimizing performance bottlenecks in your code.
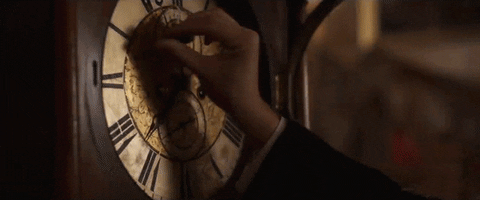
console.time('Fetching data');
fetch('https://reqres.in/api/users')
.then(response => response.json())
.then(data => {
console.timeEnd('Fetching data');
// Process the data
});
4. console.assert
This method allows you to output an error message to the console if the assertion specified in the condition is false.
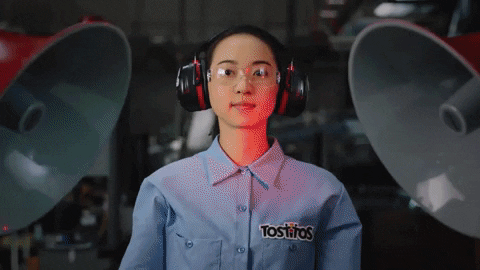
function add(a, b) {
return a + b;
}
// Test the add function
const result = add(2, 3);
console.assert(result === 5, 'Expected 2 + 3 = 5');
5. Styles for console.log
You can use the %c placeholder in your console.log statements and apply CSS styles to the console:
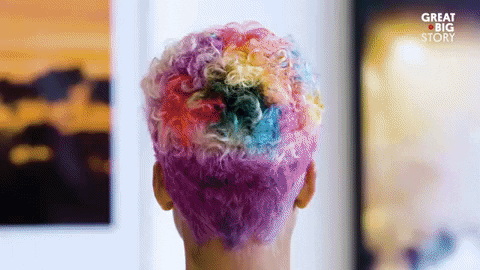
console.log('%cHello world!', 'color: red; font-weight: bold;');
6. console.trace
console.trace() is a JavaScript method that outputs the stack trace of function calls that led to the current function call. It can be used for debugging code to trace where an error or unexpected result occurred.
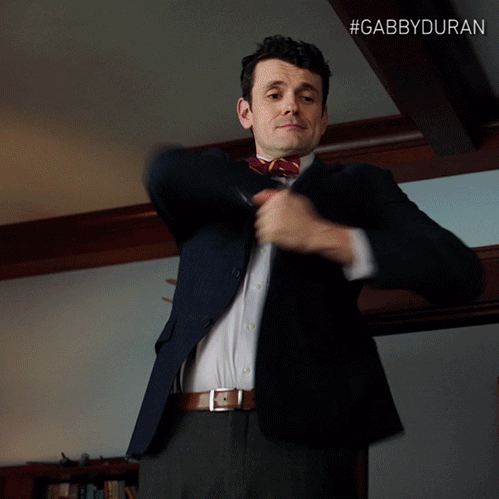
To use console.trace(), simply insert it into the code where you want to see the stack trace. For example:
function foo() {
function bar() {
console.trace();
}
bar();
}
foo();
When this code is executed, you will see the stack trace in the console starting from bar and ending at console.trace.
7. console.dir
Use the console.dir method to output an object's properties in a hierarchical format.
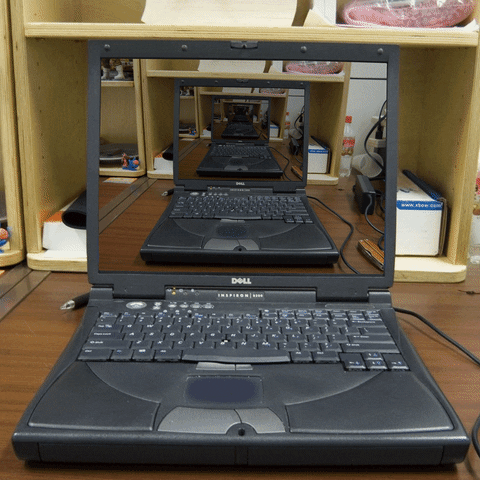
const obj = {
id: 1,
name: 'John Doe',
address: {
street: '123 Main St',
city: 'New York',
zip: 10001
}
};
console.dir(obj);
This will display the properties of the obj object in a hierarchical format, allowing you to see the object's structure and all its properties and values.
8. console.count
console.count allows you to count the number of identical messages in your code, for example.
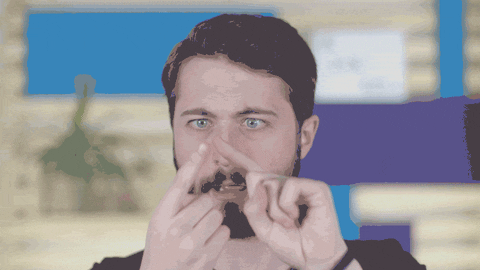
console.count('hello');
console.count('world');
console.count('hello');
9. console.clear
When you need to clear the console from unnecessary messages, you can use console.clear.
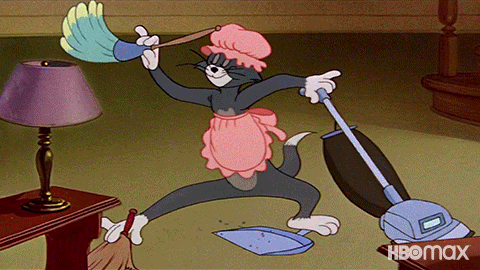
10. console.profile
console.profile is a method that you can use to profile your JavaScript code. It allows you to start profiling JavaScript code and gather information about the execution time of functions. This helps you find performance bottlenecks in your code that can be improved.
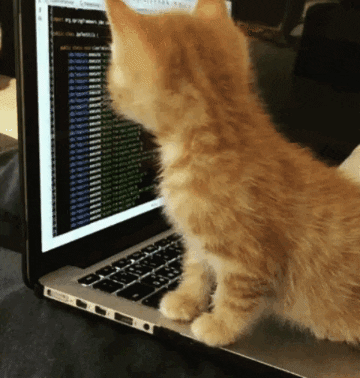
console.profile('MyProfile');
// Run some code that you want to measure the performance of
for (let i = 0; i < 100000; i++) {
// Do something
}
console.profileEnd('MyProfile');
The console will output the following information: